티스토리 뷰
ViewGroup
여러 View들을 포함할 수 있는 컨테이너 클래스, 여러 View를 담아 레이아웃을 구성하는 컨테이너
ViewGroup의 종류
LinearLayout
- 자식 뷰를 수평(가로) 또는 수직(세로)으로 순차적으로 정렬하는 레이아웃
Spinner
- 사용자가 선택할 수 있는 드롭다운 메뉴를 제공하는 AdapterView 기반의 위젯
- 단일 선택 항목 리스트를 보여주며, 사용자가 선택한 값 반환
ScrollView
- 화면 크기를 초과하는 콘텐츠를 스크롤 가능하게 만드는 레이아웃
- 기본적으로 자식 뷰는 하나만 포함 가능, 여러 뷰를 배치하려면 레이아웃(예: LinearLayout)으로 감싸야 함
- 수직 스크롤만 지원하며, 수평 스크롤을 원하면 HorizontalScrollView를 사용해야 함
RelativeLayout
- 자식 뷰를 서로 상대적인 위치 또는 부모 컨테이너의 위치를 기준으로 배치하는 레이아웃
- 뷰 간 관계를 설정할 수 있는 속성 사용:
- layout_below: 다른 뷰의 아래에 배치
- layout_toRightOf: 다른 뷰의 오른쪽에 배치
- layout_alignParentTop: 부모의 상단에 정렬
RadioGroup
- RadioButton을 포함하는 그룹으로, 단일 선택 기능 제공
- 동일 그룹 내에서 하나의 항목만 선택 가능
- 방향(수평 또는 수직)을 설정할 수 있음
추가적인 ViewGroup 종류
- ConstraintLayout: 복잡한 UI를 효율적으로 배치할 수 있는 강력한 레이아웃.
- FrameLayout: 자식 뷰를 겹쳐서 배치할 때 사용.
- TableLayout: 자식 뷰를 테이블 구조로 배치.
- GridLayout: 자식 뷰를 그리드 형태로 배치.
Spinner 화면 구성
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:gravity="center_horizontal"
android:padding="50dp">
<Spinner
android:id="@+id/spinner"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
style="@style/Widget.AppCompat.Spinner.Underlined"/>
</LinearLayout>
MainActivity.java
package com.example.test;
import android.os.Bundle;
import android.widget.ArrayAdapter;
import android.widget.Spinner;
import androidx.appcompat.app.AppCompatActivity;
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
String city[] = {"서울", "부산", "울산"};
Spinner s = findViewById(R.id.spinner);
ArrayAdapter a = new ArrayAdapter(this, android.R.layout.simple_spinner_item, city);
s.setAdapter(a);
}
}
Spinner 토스트 메시지 출력
MainActivity.java
- OnItemSelectedListener: 사용자가 항목을 선택하거나 변경했을 때 호출, 기존에 선택된 항목이 다시 클릭되어도 호출되지 않을 수 있음
package com.example.test;
import android.os.Bundle;
import android.view.View;
import android.widget.AdapterView;
import android.widget.ArrayAdapter;
import android.widget.Spinner;
import android.widget.Toast;
import androidx.appcompat.app.AppCompatActivity;
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
String city[] = {"서울", "부산", "울산"};
Spinner s = findViewById(R.id.spinner);
ArrayAdapter a = new ArrayAdapter(this, android.R.layout.simple_spinner_item, city);
s.setAdapter(a);
s.setOnItemSelectedListener(new AdapterView.OnItemSelectedListener(){
@Override
public void onItemSelected(AdapterView<?> adapterView, View view, int i, long l) {
Toast.makeText(MainActivity.this, city[i], Toast.LENGTH_SHORT).show();
}
@Override
public void onNothingSelected(AdapterView<?> adapterView) {
// 선택된 항목이 없을 때 처리 (필요 시 구현)
}
});
}
}
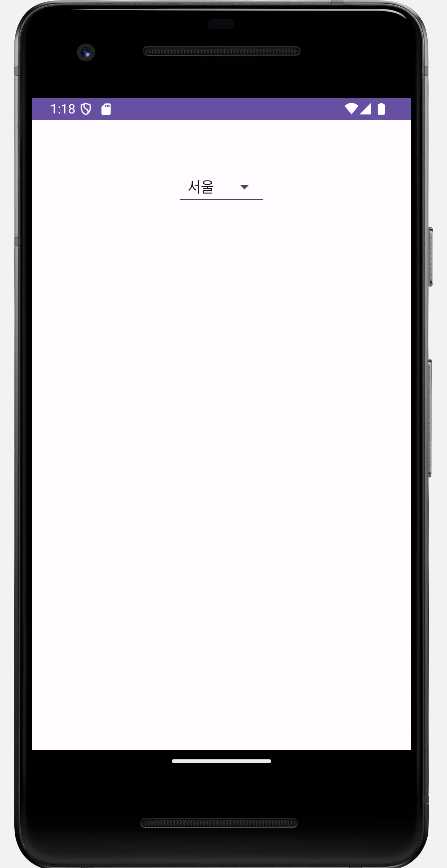

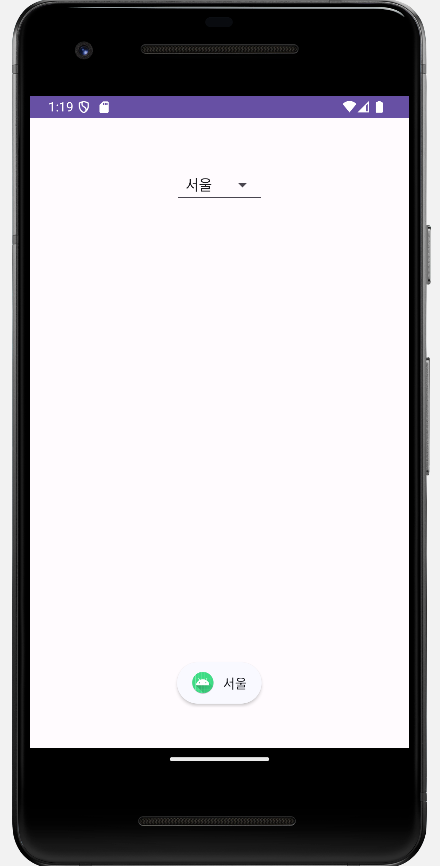
ScrollView
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<ScrollView xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:gravity="center_horizontal"
android:orientation="vertical">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:padding="40dp"
android:text="신청서 작성"
android:textSize="20sp" />
<EditText
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_margin="20dp"
android:hint="이름" />
<EditText
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_margin="20dp"
android:hint="국적" />
<EditText
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_margin="20dp"
android:hint="거주지(도시명 등)" />
<EditText
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_margin="20dp"
android:hint="상세주소" />
<EditText
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_margin="20dp"
android:hint="전자메일주소" />
<EditText
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_margin="20dp"
android:hint="연락처" />
<EditText
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_margin="20dp"
android:hint="비상 연락처 (선택)" />
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="제출" />
</LinearLayout>
</ScrollView>
MainActivity.java
package com.example.test;
import android.os.Bundle;
import androidx.appcompat.app.AppCompatActivity;
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
}
}
ScrollView (no XML)
MainActivity.java
package com.example.test;
import android.os.Bundle;
import android.view.Gravity;
import android.view.ViewGroup;
import android.widget.Button;
import android.widget.EditText;
import android.widget.LinearLayout;
import android.widget.ScrollView;
import android.widget.TextView;
import androidx.appcompat.app.AppCompatActivity;
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
ScrollView s = new ScrollView(this);
s.setLayoutParams(new ViewGroup.LayoutParams(ViewGroup.LayoutParams.MATCH_PARENT, ViewGroup.LayoutParams.MATCH_PARENT));
LinearLayout ll = new LinearLayout(this);
ll.setLayoutParams(new LinearLayout.LayoutParams(ViewGroup.LayoutParams.MATCH_PARENT, ViewGroup.LayoutParams.WRAP_CONTENT));
ll.setGravity(Gravity.CENTER_HORIZONTAL);
ll.setOrientation(LinearLayout.VERTICAL);
TextView tv=new TextView(this);
tv.setLayoutParams(new LinearLayout.LayoutParams(ViewGroup.LayoutParams.WRAP_CONTENT, ViewGroup.LayoutParams.WRAP_CONTENT));
int px=dp2px(40);
tv.setPadding(px,px,px,px);
tv.setTextSize(20);
tv.setText("신청서 작성");
ll.addView(tv);
String v[]={"이름", "국적", "거주지(도시명 등)", "상세주소", "전자메일주소", "연락처", "비상연락처 (선택)"};
px=dp2px(20);
for (int i = 0; i < 7; i++) {
EditText edittext = new EditText(this);
LinearLayout.LayoutParams lp=new LinearLayout.LayoutParams(ViewGroup.LayoutParams.MATCH_PARENT, ViewGroup.LayoutParams.WRAP_CONTENT);
lp.setMargins(px, px, px, px);
edittext.setLayoutParams(lp);
edittext.setHint(v[i]);
ll.addView(edittext);
}
Button button=new Button(this);
button.setLayoutParams(new LinearLayout.LayoutParams(ViewGroup.LayoutParams.WRAP_CONTENT, ViewGroup.LayoutParams.WRAP_CONTENT));
button.setText("제출");
ll.addView(button);
s.addView(ll);
setContentView(s);
}
private int dp2px(int dp) { return (int)(dp*getResources().getDisplayMetrics().density+0.5); }
}
'학업 > 모바일프로그래밍' 카테고리의 다른 글
[Android Studio] XML inflater 활용: 다중 화면 처리 (1) | 2024.12.15 |
---|---|
[Android Studio] 기차표 예약 화면 구현 (0) | 2024.12.15 |
[Android Studio] 배경색 랜덤 변경 및 로그캣(콘솔)/토스트 메시지 출력 (no Xml) (1) | 2024.12.14 |
[Android Studio] View, 연료 유형 선택 폼, 주문 내역 작성 폼 화면 구성 (0) | 2024.12.14 |
[Android Studio] xml로 화면에 동서남북 출력하기 (0) | 2024.11.30 |
공지사항
링크